Validation & Verification (Cambridge (CIE) O Level Computer Science): Revision Note
Exam code: 2210
Validation
What is validation?
Validation is an automated process where a computer checks if a user input is sensible and meets the program's requirements.
There are six categories of validation which can be carried out on fields and data types, these are
Range check
Length check
Type check
Presence check
Format check
Check digit
There can be occasions where more than one type of validation will be used on a field
An example of this could be a password field which could have a length, presence and type check on it
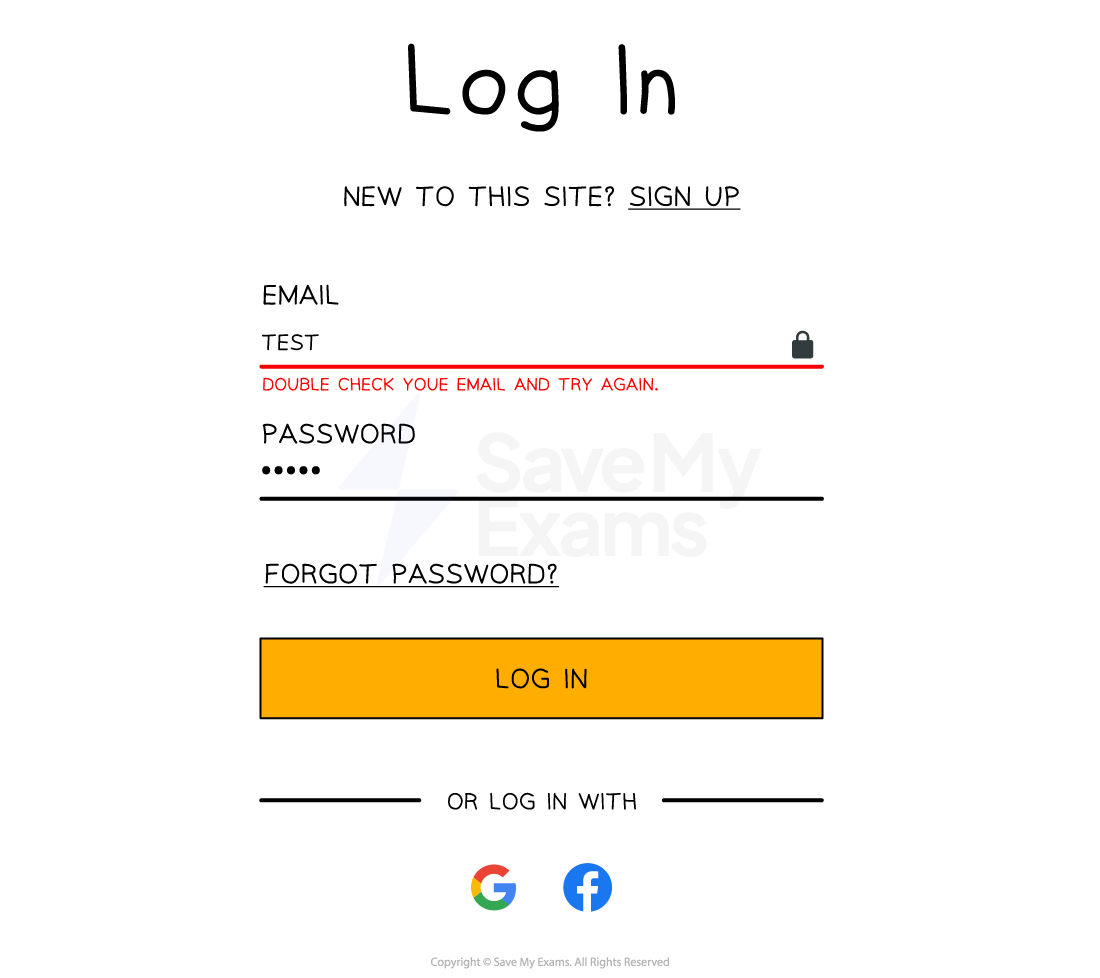
Range check
Ensures the data entered as a number falls within a particular range
Pseudocode | Ensures a percentage number is between 0-100 inclusive |
---|---|
|
Python | Ensures a user's age has been entered and falls between the digits of 0-110 inclusive |
---|---|
|
Length check
Checks the length of a string
Pseudocode | Ensures a pin number can only contain 4 digits |
---|---|
|
Python | Ensures a password is 8 characters or more |
---|---|
|
Type check
Check the data type of a field
Pseudocode | Ensures an age should be entered as an integer (whole number) |
---|---|
|
Python | Ensures an age should be entered as an integer (whole number) |
---|---|
|
Presence check
Looks to see if any data has been entered in a field
Pseudocode | Ensures username and password are both entered |
---|---|
|
Python | Ensures when registering for a website the name field is not left blank |
---|---|
|
Format check
Ensures that the data has been entered in the correct format
Format checks are done using pattern matching and string handling
Pseudocode | Ensures a six digit ID number is entered against the format "XX9999" where X is an uppercase alphabetical letter and 9999 is a four digit number |
---|---|
| |
Explanation | |
|
Python | Ensures an email contains a '@' and ' full stop (.) which follows the format "[email protected]" |
---|---|
|
Check digits
Check digits are numerical values that are the final digit of a larger code such as a barcode or an International Standard Book Number (ISBN)
They are calculated by applying an algorithm to the code and are then attached to the overall code
Verification
What is verification?
Verification is the act of checking data is accurate when entered into a system
Mistakes such as creating a new account and entering a password incorrectly mean being locked out of the account immediately
Verification methods include:
double entry checking
visual checks
Double entry checking
Double entry checking involves entering the data twice in separate input boxes and then comparing the data to ensure they both match
If they do not, an error message is shown
Pseudocode | |
---|---|
|
Visual checks
Visual checks involve the user visually checking the data on the screen
A popup or message then asks if the data is correct before proceeding
If it isn’t the user then enters the data again
Pseudocode | |
---|---|
|
Worked Example
Describe the purpose of validation and verification checks during data entry. Include an example for each.
[4]
Answers
Validation check
[1] for description:
To test if the data entered is possible / reasonable / sensible
A range check tests that data entered fits within specified values
[1] for example:
Range / length / type / presence / format
Verification check
[1] for description:
To test if the data input is the same as the data that was intended to be input
A double entry check expects each item of data to be entered twice and compares both entries to check they are the same
[1] for example:
Visual / double entry
Ready to test your students on this topic?
- Create exam-aligned tests in minutes
- Differentiate easily with tiered difficulty
- Trusted for all assessment types
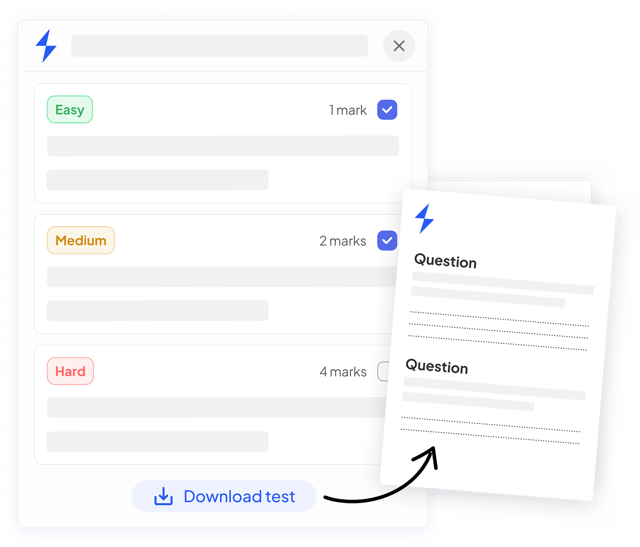
Did this page help you?