5 Sorting & Searching Algorithm Lesson Activities for GCSE
Written by: Robert Hampton
Reviewed by: James Woodhouse
Last updated
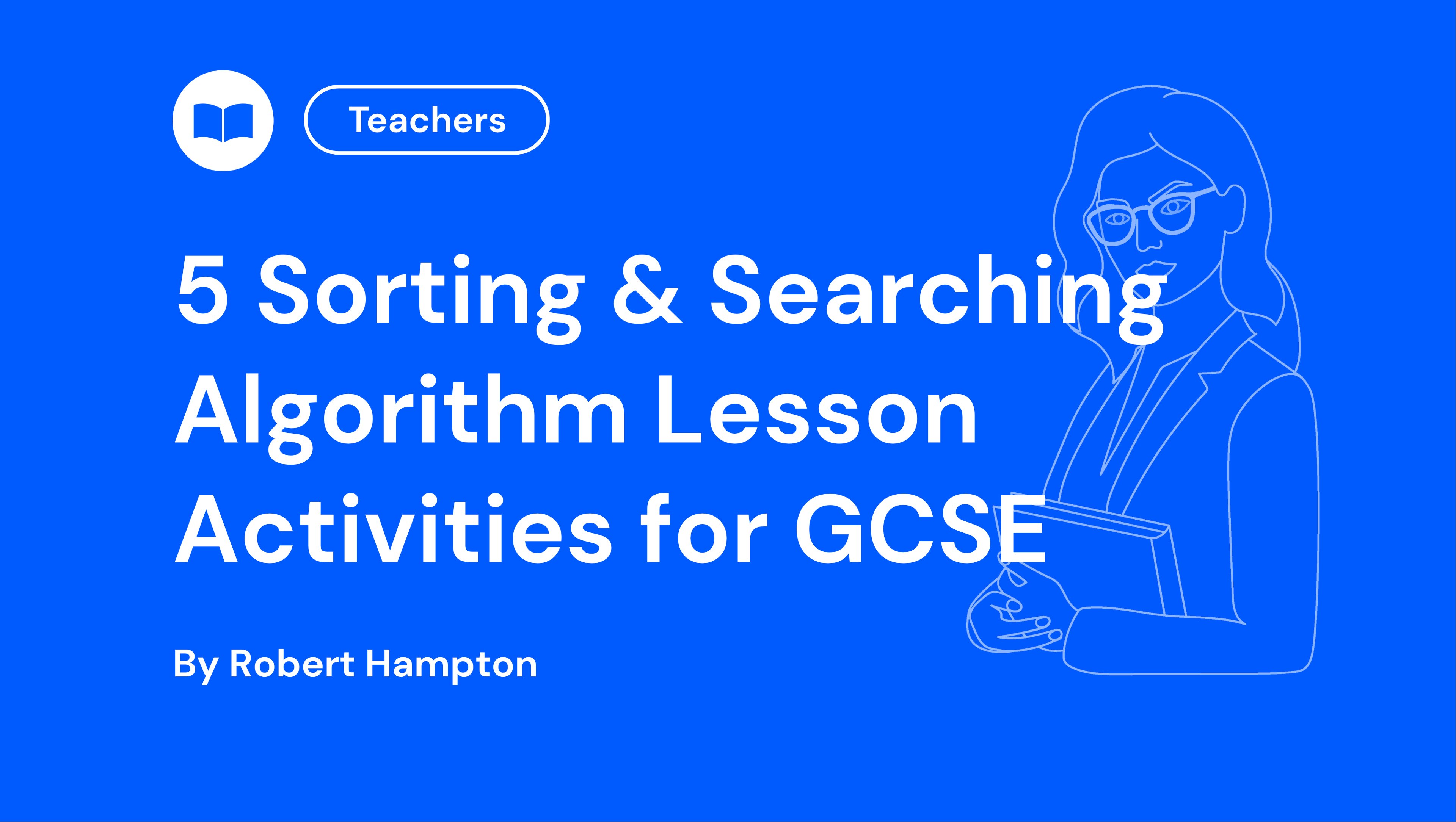
Contents
Understanding sorting and searching algorithms is essential for GCSE Computer Science students. These hands-on activities reinforce key concepts, from linear and binary search to bubble, insertion, and merge sort.
Beginner Activities: Building Algorithm Foundations
These activities introduce the core principles of sorting and searching algorithms in an engaging way.
1. Sorting Race
Objective: Help students understand the differences in efficiency between sorting algorithms.
How to do it:
Give students sets of shuffled playing cards or numbered flashcards
Assign each group a different sorting algorithm (bubble sort, insertion sort, or merge sort)
Ask them to sort their cards following the steps of their assigned algorithm
Time each group to compare the speed of different sorting methods
Example: A group assigned bubble sort repeatedly swaps adjacent cards until the list is sorted, while another group using merge sort divides the cards into smaller groups before combining them in order.
Reflection Questions:
Which sorting algorithm was the fastest? Why?
How does the number of comparisons and swaps affect performance?
Why is this technique effective?
This activity provides a tangible, interactive way for students to see how different sorting methods work and why some are more efficient than others.
For students, they get:
A practical understanding of sorting algorithms
First-hand experience of time complexity differences
Improved confidence in recognising sorting techniques in exam questions
This technique prepares students for exam-style questions such as Question 8b, which asks, “Explain one advantage of a merge sort compared to a bubble sort.” Understanding sorting speeds and trade-offs is crucial for tackling algorithm-related exam questions.
2. Linear vs Binary Search Challenge
Objective: Teach students the difference between linear and binary search.
How to do it:
Prepare a list of random numbers (printed or displayed digitally)
Choose a target number and have one student use linear search (checking each number sequentially)
Have another student use binary search, ensuring the list is sorted first and using the divide-and-conquer method
Compare the number of steps taken in each method
Example: Searching for the number 42 in a list of 100 numbers:
A linear search may take up to 100 steps in the worst-case
A binary search will take at most 7 steps (log₂ 100 ≈ 7)
Reflection Questions:
Why does binary search require a sorted list?
In what situations is linear search more effective?
Why is this technique effective?
This hands-on activity reinforces why binary search is significantly faster than linear search for large datasets.
For students, they get:
A clear comparison of search efficiencies
An intuitive understanding of divide-and-conquer
Confidence in applying search techniques in exam scenarios
This activity supports exam-style questions such as: “Explain why binary search is more efficient than linear search for large datasets.” By performing both searches themselves, students build a strong understanding of search efficiency.
Intermediate Activities: Applying Sorting and Searching Algorithms
These activities require students to apply their knowledge to solve algorithm problems.
3. Pseudocode Sorting Challenge
Objective: Strengthen students' ability to write and interpret sorting algorithms in pseudocode.
How to do it:
Provide students with scrambled lines of pseudocode representing sorting algorithms (bubble sort, insertion sort, merge sort)
Ask them to arrange the lines in the correct order
Challenge them to modify the pseudocode to handle edge cases (e.g., sorting an already sorted list)
Example: A scrambled pseudocode for insertion sort requires students to correctly sequence the loop structure and comparisons.
Prepare Scrambled Pseudocode:
Provide students with scrambled pseudocode for insertion sort
The sorting algorithm is broken into disordered steps that students must rearrange correctly
WHILE j >= 0 AND array[j] > key
j = j - 1
FOR i FROM 1 TO length(array) - 1
key = array[i]
array[j + 1] = key
array[j + 1] = array[j]
END FOR
j = i - 1
END WHILE
Arrange the Lines in the Correct Order:
Students work in pairs or small groups
They must rearrange the scrambled pseudocode to form a correctly structured algorithm
Encourage them to test the logic by walking through an example with a small list
FOR i FROM 1 TO length(array) - 1
key = array[i]
j = i - 1
WHILE j >= 0 AND array[j] > key
array[j + 1] = array[j]
j = j - 1
END WHILE
array[j + 1] = key
END FOR
Modify the Pseudocode for Edge Cases:
Once the students have the correct order, challenge them to modify the pseudocode to handle edge cases, such as:
An already sorted list
A list with duplicate elements
A list containing only one element
Reflection Questions:
How does the logic of each sorting algorithm differ in pseudocode?
What steps can be removed or optimised in sorting algorithms?
Why is this technique effective?
This activity bridges the gap between understanding the algorithm and writing correct code.
For students, they get:
Practical experience with pseudocode structuring
Improved debugging and logical thinking skills
A deeper understanding of sorting mechanics
This technique helps with exam questions like: “Write pseudocode for an insertion sort algorithm.” Practising with scrambled pseudocode strengthens students’ ability to recall and write sorting algorithms from memory.
4. Sorting Algorithm Debugging
Objective: Develop students' skills in identifying and fixing errors in sorting algorithms.
How to do it:
Provide students with faulty sorting algorithm code (Python or pseudocode) containing intentional errors
Ask them to identify and correct the mistakes
Discuss how each error affects the algorithm’s performance
Example: A bubble sort implementation that incorrectly swaps elements or has an off-by-one error in the loop index.
def bubble_sort(arr):
n = len(arr)
for i in range(n): # Error: Should be range(n-1)
for j in range(n-1): # Error: Should be range(n-i-1)
if arr[j] < arr[j+1]: # Error: Should be '>' for ascending order
temp = arr[j]
arr[j] = arr[j+1]
arr[j+1] = temp
return arr
The outer loop runs one extra iteration, leading to unnecessary comparisons
The inner loop does not reduce in size, making the algorithm inefficient
The comparison is < instead of >, causing incorrect sorting
def bubble_sort(arr):
n = len(arr)
for i in range(n-1): # Fixed loop range
for j in range(n-i-1): # Stops unnecessary comparisons
if arr[j] > arr[j+1]: # Corrected condition for ascending order
arr[j], arr[j+1] = arr[j+1], arr[j] # Swaps correctly
return arr
Reflection Questions:
What common mistakes can occur in sorting algorithms?
How do we test if a sorting algorithm works correctly?
Why is debugging important in algorithm design?
Why is this technique effective?
Debugging sorting algorithms builds critical thinking and problem-solving skills.
For students, they get:
Hands-on experience debugging real code
A structured approach to testing sorting algorithms
A deeper understanding of common logic errors
This activity prepares students for code analysis questions in exams, such as: “Identify and correct the error in the given sorting algorithm.” By debugging real examples, students build problem-solving confidence.
Advanced Activities: Deepening Algorithm Understanding
These activities challenge students to think critically about sorting and searching algorithms.
5. Real-World Algorithm Applications
Objective: Show students how sorting and searching algorithms are used in real-world applications.
How to do it:
Discuss examples of sorting and searching in technology (e.g., search engines, databases, recommendation systems)
Ask students to analyse a real-world scenario and propose an appropriate algorithm
Example: How does Google use sorting algorithms to rank web pages? When would binary search be used in a database lookup?
Discuss Real-World Examples:
Explain how sorting and searching algorithms power everyday technology:
Search engines (e.g., Google sorts results based on relevance)
Databases (e.g., searching for customer records efficiently)
E-commerce websites (e.g., sorting products by price or rating)
Social media feeds (e.g., ranking posts by engagement)
Scenario Analysis:
Present students with real-world problems and ask them to choose an appropriate algorithm to solve them
Students should justify their choice and explain how the algorithm works
Example Scenarios:
Online Shopping Search: "A website lets users search for products by name. Which search algorithm should be used and why?"
Hospital Database: "A hospital database stores thousands of patient records. How can a search algorithm be used to quickly find a patient by surname?"
Video Streaming Services: "Netflix recommends content based on user preferences. What sorting method could help prioritise the most relevant movies?"
Class Discussion & Justification:
Groups present their chosen algorithms and explain their reasoning
Compare solutions and discuss why some algorithms work better for certain tasks
Example Answer:
Scenario: "An online bookstore needs to display books sorted by customer ratings."
Algorithm Choice: Merge Sort (efficient for large lists, stable sorting keeps books with the same rating in the same order)
Reflection Questions:
How do companies use sorting and searching algorithms to improve efficiency?
Why are efficient algorithms critical in large-scale applications?
Why is this technique effective?
It helps students connect algorithm theory to practical applications in computing and technology.
For students, they get:
A better understanding of how sorting and searching impact technology
The ability to analyse real-world problems and propose algorithmic solutions
Confidence in applying knowledge to exam questions such as:
"Explain why binary search is used in databases instead of linear search."
"Describe how sorting algorithms are used in real-world applications."
Conclusion
By engaging in these hands-on activities, students will develop a strong understanding of sorting and searching algorithms. These practical exercises prepare them for exam success and future applications in computing and software development.
Sign up for articles sent directly to your inbox
Receive news, articles and guides directly from our team of experts.
Share this article