College Board AP Computer Science Units: Full List
Written by: Robert Hampton
Reviewed by: James Woodhouse
Last updated
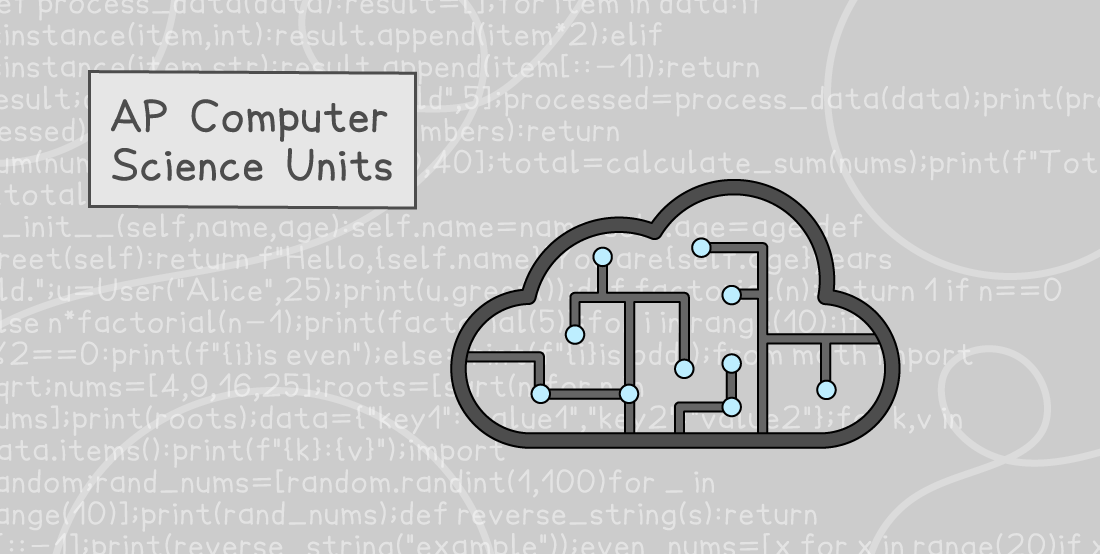
Contents
AP Computer Science consists of two courses, each with a distinct focus while sharing some common principles.
College Board AP Computer Science Principles (CSP) explores broad concepts, including data analysis, how the Internet works, and the impact of computer science in fields such as cybersecurity and artificial intelligence.
College Board AP Computer Science A (CSA), on the other hand, focuses on programming with Java, covering complex techniques used to develop software.
In this article, I’ll break down each course, providing an overview of the topics covered in each unit to help you organise your studies effectively.
College Board AP Computer Science Principles (CSP) Units
1. Creative Development
This topic explores how programs are designed, developed, and refined to meet specific needs. You will learn the importance of collaboration in programming, how to define a program’s purpose, and how to structure code for readability and efficiency. Additionally, you will develop skills in debugging and testing to identify and correct errors, ensuring that programs function as intended. Ethical computing practices, including intellectual property rights and citation, are also covered in responsible computing.
Topics include:
The role of collaboration in program development
Investigating a problem and defining its purpose
Designing effective solutions and structuring code
Debugging and testing to identify and correct errors
Ethical computing practices, including intellectual property rights and citation
2. Data
This topic explores how computers represent, manipulate, and analyze data. You will learn about binary number systems, data compression techniques, and how programs can extract meaningful insights from data. Understanding how data is structured and processed is essential for making informed decisions and solving problems effectively. Additionally, you will examine the ethical implications of data collection and its impact on society.
Topics include:
How computers use binary to store and process data
Data compression methods and their effects on storage and transmission
Extracting useful information from large datasets
Implementing algorithms to process and analyze data
Ethical considerations and the societal impact of data collection
3. Algorithms and Programming
This topic introduces you to the fundamental principles of programming and algorithm development. You will learn how to use variables, conditionals, loops, and functions to write efficient code. The course also covers abstraction, procedural programming, and key computational techniques such as iteration, searching, and algorithmic efficiency. These concepts will help you break down complex problems into manageable solutions and write programs that solve real-world challenges.
Topics include:
Variables, data abstraction, and expressions
Boolean logic, conditionals, and loops
Developing and evaluating algorithms
Lists, binary search, and procedure calls
Using simulations and evaluating algorithm efficiency
4. Computer Systems and Networks
This topic explores the structure and function of computer systems, including how they connect and communicate over networks. You will learn about the internet, fault tolerance in computing systems, and how parallel and distributed computing improves efficiency. Understanding these concepts will help you grasp how modern computing infrastructure operates and supports various applications.
Topics include:
The internet and how computing systems work
Ensuring system reliability through fault tolerance
Parallel and distributed computing for improved performance
5. Impact of Computing
This topic examines the influence of computing on society, from its benefits and challenges to ethical and legal considerations. You will explore issues like the digital divide, computing bias, and the role of crowdsourcing, while also evaluating the ethical responsibilities of technology use. Safe computing practices, including personal data protection and cybersecurity best practices, are also key areas of focus.
Topics include:
Beneficial and harmful effects of computing
The digital divide and accessibility challenges
Computing bias and responsible technology use
Crowdsourcing and collaboration in technology
Legal and ethical responsibilities in computing
Safe computing and data security, including personal data protection and cybersecurity best practices
What is Covered in the College Board AP Computer Science Principles (CSP) Exam?
Section | Question Type/Component | Number of Questions | Exam Weighting | Timing |
I | Multiple-choice questions | 70 | 70% | 120 minutes End-of-course |
Single-select | 57 | |||
Segle-select with a reading passage about computing innovation | 5 | |||
Multi-select | 8 | |||
II | Create Performance Task | 30% | ||
Program code, video, and Personalized Project Reference | At least 9 hours in class | |||
Written response questions related to the Create Performance task | 2 | 60 minutes End-of-course AP Exam |
Units | Exam Weighting |
| 10-13% |
| 17-22% |
| 30-35% |
| 11-15% |
| 21-26% |
Revision Resources for College Board AP Computer Science Principles (CSP)
For high-quality revision resources for the College Board AP Computer Science Principles (CSP) exam, explore our revision resources.
College Board AP Computer Science A (CSA) Units
1. Primitive Types
This topic introduces you to fundamental programming concepts in Java, including variables, data types, and expressions. You will learn how to declare and manipulate variables, use arithmetic and compound assignment operators, and understand typecasting. These concepts form the foundation for writing effective Java programs.
Topics include:
The purpose of programming and why Java is widely used
Declaring and using variables and data types
Writing expressions and assignment statements
Using compound assignment operators for efficiency
Type casting and understanding variable range limits
2. Using Objects
This topic focuses on working with objects in Java, a key aspect of object-oriented programming. You will learn how to create and store objects, call methods, and manipulate data using wrapper classes and built-in Java libraries. These skills will help you understand how to interact with and utilize objects effectively in your programs.
Topics include:
Understanding objects as instances of classes
Creating and storing objects through instantiation
Calling void and non-void methods with and without parameters
Working with string objects and methods
Using wrapper classes like Integer and Double
Performing mathematical operations with the Math class
3. Boolean Expressions and If Statements
This topic introduces Boolean logic and decision-making in Java programs. You will learn how to construct Boolean expressions, control the flow of a program using if, if-else, and else if statements, and compare objects effectively. Understanding these concepts will allow you to write programs that make decisions based on user input and other conditions.
Topics include:
Constructing and evaluating Boolean expressions
Using if, if-else, and else if statements for decision-making
Writing compound Boolean expressions for complex conditions
Determining equivalency between different Boolean expressions
Comparing objects using Java methods
4. Iteration
This topic covers loops and repetition structures in Java, enabling you to write programs that execute blocks of code multiple times. You will learn to use while and for loops, develop algorithms that manipulate strings, and apply nested iteration for more complex tasks. Additionally, you will analyze code execution and predict how changes impact program behavior.
Topics include:
Using while loops to repeat tasks based on conditions
Implementing for loops for controlled iteration
Developing algorithms using loops and string manipulation
Writing and understanding nested iteration for more advanced patterns
Analyzing code execution to determine the number of iterations
5. Writing Classes
This topic introduces object-oriented programming by guiding you through the structure and functionality of Java classes. You will learn how to design and implement classes, use constructors to initialize objects, and define methods to manipulate data. Additionally, you will explore the use of static variables and methods to manage shared resources efficiently.
Topics include:
Understanding the anatomy of a Java class
Writing constructors to initialize objects
Documenting code with comments for clarity
Implementing accessor and mutator methods
Creating methods to define behaviors
Utilizing static variables and methods for shared functionality
6. Arrays
This topic focuses on how to create, manipulate, and traverse arrays in Java. You will learn how to initialize arrays, access their elements, and iterate through them using loops. Additionally, you will explore enhanced for loops and develop algorithms that utilize arrays to store and process data efficiently.
Topics include:
Creating and accessing elements in arrays
Traversing arrays using loops
Using enhanced for loops for array traversal
Developing algorithms that utilize arrays effectively
7. ArrayLists
This topic introduces ArrayLists, a dynamic data structure that allows flexible storage and manipulation of objects in Java. You will learn how to initialize and modify ArrayLists, explore built-in methods, and traverse them using loops. Additionally, you will develop algorithms that utilize ArrayLists for searching and sorting, while considering ethical issues surrounding data collection.
Topics include:
Initializing and using ArrayLists
Utilizing ArrayList methods for manipulation
Traversing ArrayLists using loops
Developing algorithms using ArrayLists
Searching and sorting techniques
Ethical concerns related to data collection
8. 2D Arrays
This topic expands on arrays by introducing two-dimensional (2D) arrays, which allow data to be organized in a grid-like structure. You will learn how to declare, initialize, and manipulate 2D arrays, as well as traverse them using loops. Additionally, you will develop algorithms that work with 2D arrays and apply test cases to validate results.
Topics include:
Declaring and initializing 2D arrays
Accessing and modifying elements in a 2D array
Traversing 2D arrays using nested loops
Developing algorithms for 2D arrays
Validating results using test cases
9. Inheritance
This topic explores how object-oriented programming enables code reuse and hierarchy through inheritance. You will learn how to create superclasses and subclasses, override methods, and use the super keyword to reference parent class functionality. Additionally, you will understand polymorphism, how references work in inheritance hierarchies, and the role of the Object superclass.
Topics include:
Creating superclasses and subclasses
Writing constructors for subclasses
Overriding methods in subclasses
Using the super keyword
Creating references using inheritance hierarchies
Understanding polymorphism and dynamic method dispatch
The role of the Object superclass in Java
10. Recursion
This topic explores the concept of recursion, where a method calls itself to solve a problem. You will learn how recursive algorithms work, their advantages, and when they are appropriate to use. Additionally, you will examine recursive searching and sorting techniques, understanding their efficiency and execution flow.
Topics include:
Understanding recursive methods and their structure
Tracing recursive function execution
Recursive searching and sorting algorithms
Evaluating the efficiency and limitations of recursion
What is Covered in the College Board AP Computer Science A (CSA) Exam?
Section | Question Type/Component | Number of Questions | Exam Weighting | Timing |
I | Multiple-choice questions | 40 | 50% | 90 minutes |
II | Free-response questions | 4 | 90 minutes | |
Question 1: Methods and Control Structures | 12.5% | |||
Question 2: Class | 12.5% | |||
Question 3: Array/ArrayList | 12.5% | |||
Question 4: 2D Array | 12.5% |
Units | Exam Weighting |
| 2.5-5% |
| 5-7.5% |
| 15-17.5% |
| 17.5-22% |
| 5.7.5% |
| 10-15% |
| 2.5-7.5% |
| 7.5-10% |
| 5-10% |
| 5-7.5% |
Improve Your Grades with Save My Exams
At Save My Exams, we’re here to help you achieve the best possible grade in AP Computer Science. Right now, you can access our past papers with mark schemes, giving you the perfect way to practise and refine your exam technique.
And that’s just the beginning - our expert team is working on even more resources to support your revision, including:
Clear and concise revision notes that break down complex topics into easy-to-understand explanations
Exam-style questions with detailed mark schemes, so you know exactly what examiners are looking for
Flashcards for quick-fire revision and easy recall of key facts and definitions
These new resources are coming soon, so stay tuned! In the meantime, get ahead with our AP Computer Science past papers and start building your exam confidence today.
Explore Our AP Computer Science Revision Resources
References
College Board AP Computer Science Principles Course and Exam Description (opens in a new tab)
College Board AP Computer Science A Course and Exam Description (opens in a new tab)
Sign up for articles sent directly to your inbox
Receive news, articles and guides directly from our team of experts.
Share this article