Describe what the algorithm represented by the flowchart is doing.
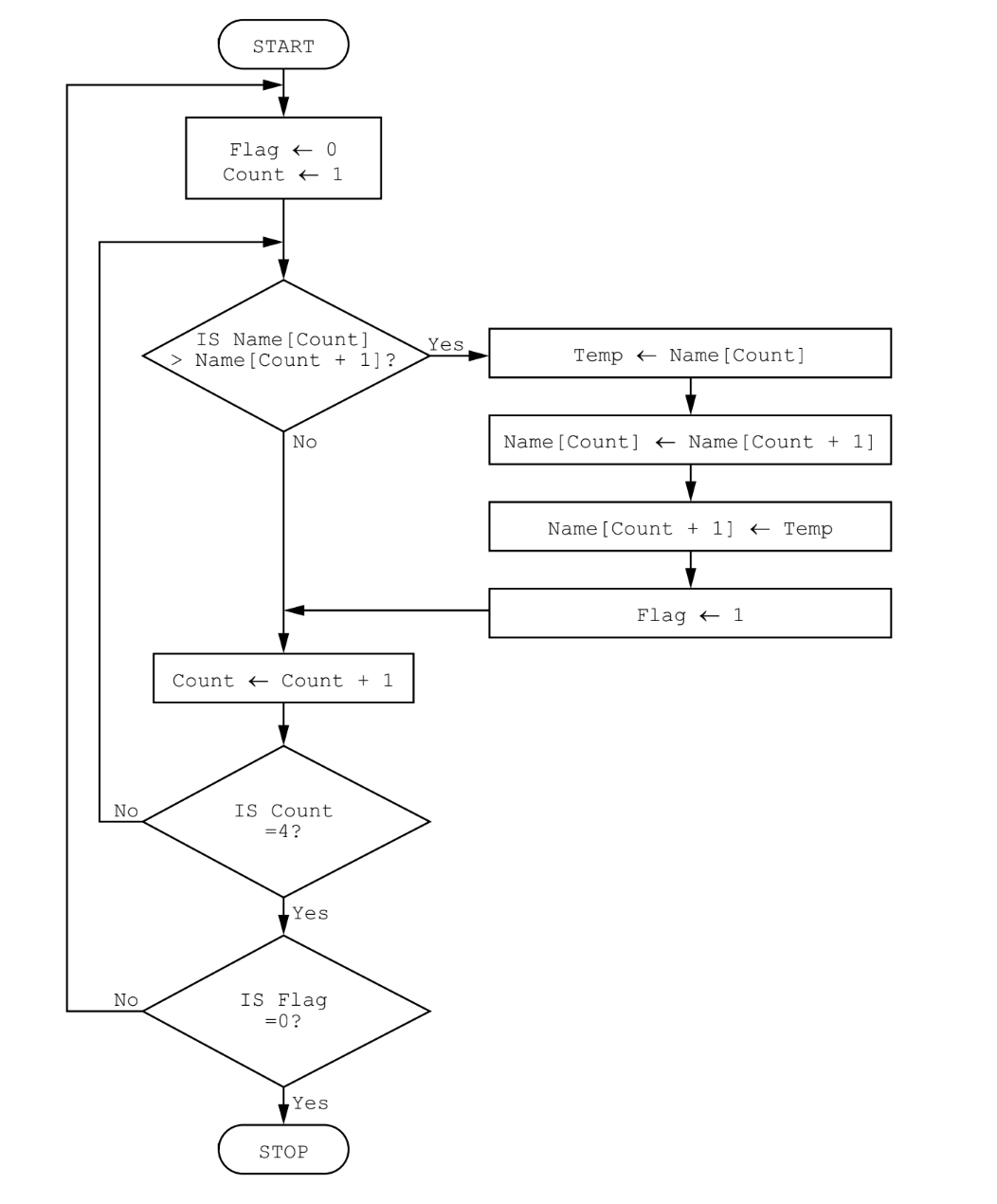
Did this page help you?
Describe what the algorithm represented by the flowchart is doing.
How did you do?
Did this page help you?
The pseudocode algorithm shown has been written by a teacher to enter marks for the students in her class and then to apply some simple processing.
Count ← 0
REPEAT
INPUT Score[Count]
IF Score[Count] >= 70
THEN
Grade[Count] ← "A"
ELSE
IF Score[Count] >= 60
THEN
Grade[Count] ← "B"
ELSE
IF Score[Count] >= 50
THEN
Grade[Count] ← "C"
ELSE
IF Score[Count] >= 40
THEN
Grade[Count] ← "D"
ELSE
IF Score[Count] >= 30
THEN
Grade[Count] ← "E"
ELSE
Grade[Count] ← "F"
ENDIF
ENDIF
ENDIF
ENDIF
ENDIF
Count ← Count + 1
UNTIL Count = 30
Describe what happens in this algorithm.
How did you do?
Did this page help you?
B ← FALSE
INPUT Num
FOR Counter ← 1 TO 12
IF A[Counter] = Num
THEN
B ← TRUE
ENDIF
NEXT Counter
Identify the purpose of the algorithm.
How did you do?
Did this page help you?
The flowchart shows an algorithm that should allow 60 test results to be entered into the variable Score
.
Each test result is checked to see if it is 50 or more. If it is, the test result is assigned to the Pass
array. Otherwise, it is assigned to the Fail
array.
Complete this flowchart
How did you do?
Write a pseudocode routine that will check that each test result entered into the algorithm is between 0 and 100 inclusive.
How did you do?
Did this page help you?
The pseudocode represents an algorithm.
The pre-defined function DIV
gives the value of the result of integer division. For example, Y = 9 DIV 4
gives the value Y = 2
The pre-defined function MOD
gives the value of the remainder of integer division. For example, R = 9 MOD 4
gives the value R = 1
First ← 0
Last ← 0
INPUT Limit
FOR Counter ← 1 TO Limit
INPUT Value
IF Value >= 100
THEN
IF Value < 1000
THEN
First ← Value DIV 100
Last ← Value MOD 10
IF First = Last
THEN
OUTPUT Value
ENDIF
ENDIF
ENDIF
NEXT Counter
Describe the purpose of the algorithm.
How did you do?
Did this page help you?
This algorithm checks the temperature of hot food being served to customers.
State how the final output from the algorithm could be improved.
How did you do?
Identify the process in the algorithm that is not required.
How did you do?
Did this page help you?
This flowchart represents an algorithm.
Describe what the algorithm represented by the flowchart is doing.
How did you do?
Did this page help you?
The variables P and Q are used to store data in a program. P stores a string. Q stores a character.
Give the value of Position after the algorithm has been executed with the data in the below question.
Write pseudocode statements to declare the variables P and Q, store "The world" in P and store 'W' in Q
How did you do?
Did this page help you?
Describe the purpose of the algorithm.
How did you do?
Did this page help you?
The variables X, Y and Z are used to store data in a program:
X stores a string
Y stores a position in the string (e.g. 2)
Z stores the number of characters in the string.
The function LENGTH(X)
finds the length of a string X.
The function SUBSTRING(X,Y,Z)
finds a substring of X starting at position Y and
Z characters long. The first character in X is in position 1.
Write pseudocode statements to:
store the string "Programming is fun
" in X
find the length of the string and output it
extract the word fun
from the string and output it.
How did you do?
Did this page help you?
The one-dimensional (1D) array StudentName[]
contains the names of students in a class. The two-dimensional (2D) array StudentMark[]
contains the mark for each subject, for each student. The position of each student’s data in the two arrays is the same, for example, the student in position 10 in StudentName[]
and StudentMark[]
is the same.
The variable ClassSize
contains the number of students in the class. The variable SubjectNo
contains the number of subjects studied. All students study the same number of subjects.
The arrays and variables have already been set up and the data stored.
Students are awarded a grade based on their average mark.
Average mark | Grade awarded |
---|---|
greater than or equal to 70 | distinction |
greater than or equal to 55 and less than 70 | merit |
greater than or equal to 40 and less than 55 | pass |
less than 40 | fail |
Write a program that meets the following requirements:
calculates the combined total mark for each student for all their subjects
calculates the average mark for each student for all their subjects, rounded to the nearest whole number
outputs for each student:
name
combined total mark
average mark
grade awarded
calculates, stores and outputs the number of distinctions, merits, passes and fails for the whole class.
You must use pseudocode or program code and add comments to explain how your code works.
You do not need to initialise the data in the array.
How did you do?
Did this page help you?
Draw a flowchart for an algorithm to:
allow numbers to be input
ignore any numbers that are negative
count how many numbers are positive
output the count of positive numbers when zero is input and end the algorithm.
How did you do?
Explain the changes you will make to your algorithm to also count the negative numbers.
How did you do?
Did this page help you?
A function LENGTH(X)
finds the length of a string X and a function SUBSTRING(X,Y,Z)
finds a substring of X
starting at position Y
and Z
characters long. The first character in the string is position 1.
Show the value of the variable after each pseudocode statement has been executed.
01 P ← "Computer Science"
.............................................................
02 Q ← LENGTH(P)
..................................................... ........................
03 R ← SUBSTRING(P,10,7)
.............................................................
04 S ← LENGTH(R)
......................................................................... ..
05 T ← SUBSTRING(R,1,3)
.................................................................
How did you do?
Did this page help you?
Rewrite this pseudocode algorithm using a WHILE … DO … ENDWHILE loop.
B ← FALSE
INPUT Num
FOR Counter ← 1 TO 12
IF A[Counter] = Num
THEN
B ← TRUE
ENDIF
NEXT Counter
How did you do?
Did this page help you?
The names of patients are stored in the one-dimensional (1D) array Patient[] of type string. A separate two-dimensional (2D) array Readings[] stores the latest data recorded about each patient. The array already contains the readings taken by a nurse for each patient:
temperature measured to one decimal place
pulse rate, a whole number.
Temperature readings should be in the range 31.6 to 37.2 inclusive.
Pulse readings should be in the range 55.0 to 100.0 inclusive.
The hospital number given to the patient is used for the index on both arrays, this is a value between 1 and 1000 inclusive.
When the data for a patient is checked a warning is given if any of the readings are out of range.
If both readings are out of range, then a severe warning is given.
Write a procedure, using pseudocode or program code, that meets the following requirements:
takes the hospital number as a parameter
checks if the number is valid
outputs an error message and exits the procedure if the number is not valid
if the hospital number is valid:
output the patient’s name
output ‘Normal readings’ if both the readings are within range
output ‘Warning’ and the name of the reading e.g. ‘Pulse’ if one reading is out of range
output ‘Severe warning’ and the names of the two readings ‘Pulse and temperature’ if both readings are out of range
exits the procedure.
You must use pseudocode or program code and add comments to explain how your code works.
You do not need to initialise the data in the arrays.
How did you do?
Did this page help you?
An algorithm has been written in pseudocode to allow some numbers to be input. All the positive numbers that are input are totalled and this total is output at the end. An input of 0 stops the algorithm.
01 Exit 1
02 WHILE Exit <> 0 DO
03 INPUT Number
04 IF Number > 0
05 THEN
06 Total Total + Number
07 ELSE
08 IF Number = 0
09 THEN
10 Exit 0
11 ENDIF
12 ENDIF
13 ENDWHILE
14 OUTPUT "The total value of your numbers is ", Total
Describe how you could change the corrected algorithm to record and output how many positive numbers have been included in the final total.
You do not need to rewrite the algorithm.
How did you do?
Did this page help you?