Iteration (Cambridge (CIE) IGCSE Computer Science)
Revision Note
Written by: Robert Hampton
Reviewed by: James Woodhouse
What is iteration?
Iteration is repeating a line or a block of code using a loop
Iteration can be:
Count controlled
Condition controlled
Nested
Count Controlled Loops
What is a count controlled loop?
A count controlled loop is when the code is repeated a fixed number of times (e.g. using a for loop)
A count controlled loop can be written as:
FOR <identifier> ← <value1> TO <value2><statements>
NEXT <identifier>
Identifiers must be an integer data type
It uses a counter variable that is incremented or decremented after each iteration
This can be written as:
FOR <identifier> ← <value1> TO <value2> STEP <increment><statements>
NEXT <identifier>
A detailed look at a Python FOR statement:
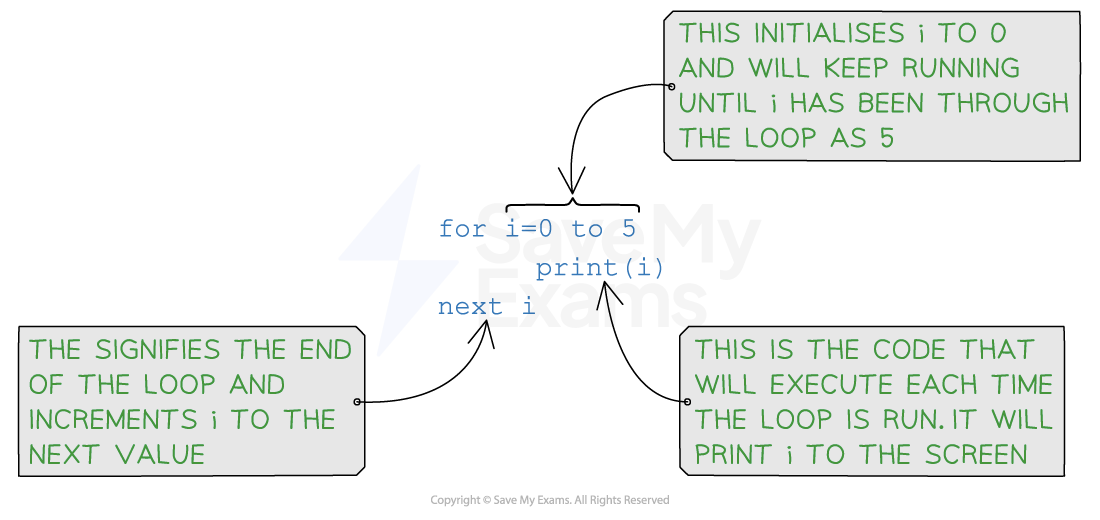
Examples
Iteration | Pseudocode | Python |
---|---|---|
Count controlled |
|
|
This will print the word "Hello" 10 times | ||
|
# Python range function excludes end value | |
This will print the even numbers from 2 to 10 inclusive | ||
|
# Python range function excludes end value | |
This will print the numbers from 10 to 0 inclusive |
Condition Controlled Loops
What is a condition controlled loop?
A condition controlled loop is when the code is repeated until a condition is met
There are two types of condition controlled loops:
Post-condition (REPEAT)
Pre-condition (WHILE)
Post-condition loops (REPEAT)
A post-condition loop is executed at least once
The condition must be an expression that evaluates to a Boolean (True/False)
The condition is tested after the statements are executed and only stops once the condition is evaluated to True
It can be written as:
REPEAT
<statement>
UNTIL <condition>
Iteration | Pseudocode | Python |
---|---|---|
Post-condition |
| # NOT USED IN PYTHON |
| # NOT USED IN PYTHON |
Pre-condition loops (WHILE)
The condition must be an expression that evaluates to a Boolean (True/False)
The condition is tested and statements are only executed if the condition evaluates to True
After statements have been executed the condition is tested again
The loop ends when the condition evaluates to False
It can be written as:
WHILE <condition> DO
<statements>
ENDWHILEA detailed look at a Python WHILE statement:
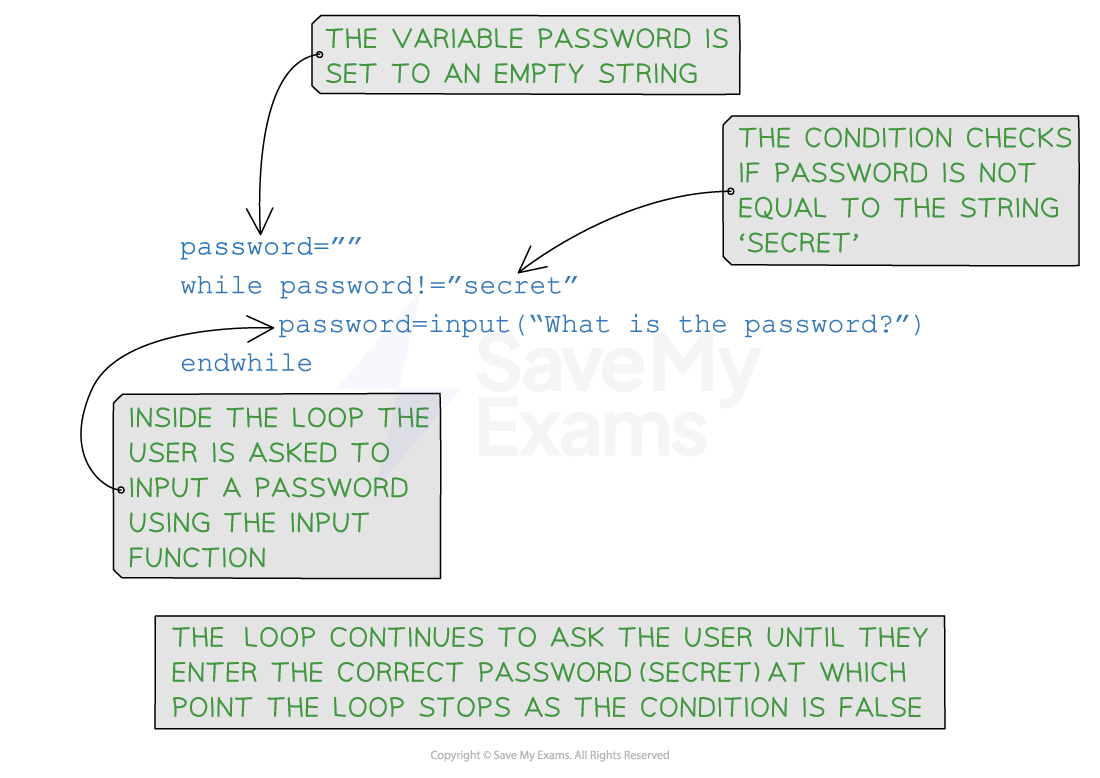
Iteration | Pseudocode | Python |
---|---|---|
Pre-condition |
|
|
|
|
Nested Iteration
What is nested selection?
Nested iteration is a loop within a loop, e.g. a FOR inside of another FOR
Nested means to be 'stored inside the other'
Example
Pseudocode |
---|
|
Python |
---|
// Program to print a multiplication table up to a given number // Prompt the user to enter a number
// Set the initial value of the counter for the outer loop
// Outer loop to iterate through the multiplication table
// Set the initial value of the counter for the inner loop // Inner loop to print the multiplication table for the current number // Calculate the product of the outer and inner counters
// Print the multiplication table entry
// Increment the inner counter
// Move to the next number in the multiplication table |
Last updated:
You've read 0 of your 10 free revision notes
Unlock more, it's free!
Did this page help you?