Programming Validation (Edexcel GCSE Computer Science) : Revision Note
Programming Validation
What is validation?
Validation is code which is used to check that an input from a user is acceptable and that it matches the requirements of the program
There are 5 main categories of validation which can be carried out on fields and data types, these are:
Length check
Range check
Presence check
Pattern check
There can be occasions where more than one type of validation will be used on a field
An example of this could be a password field which could have a length, presence and type check on it
An effective way of programming validation can be using while loops that terminate once the user has met the criteria
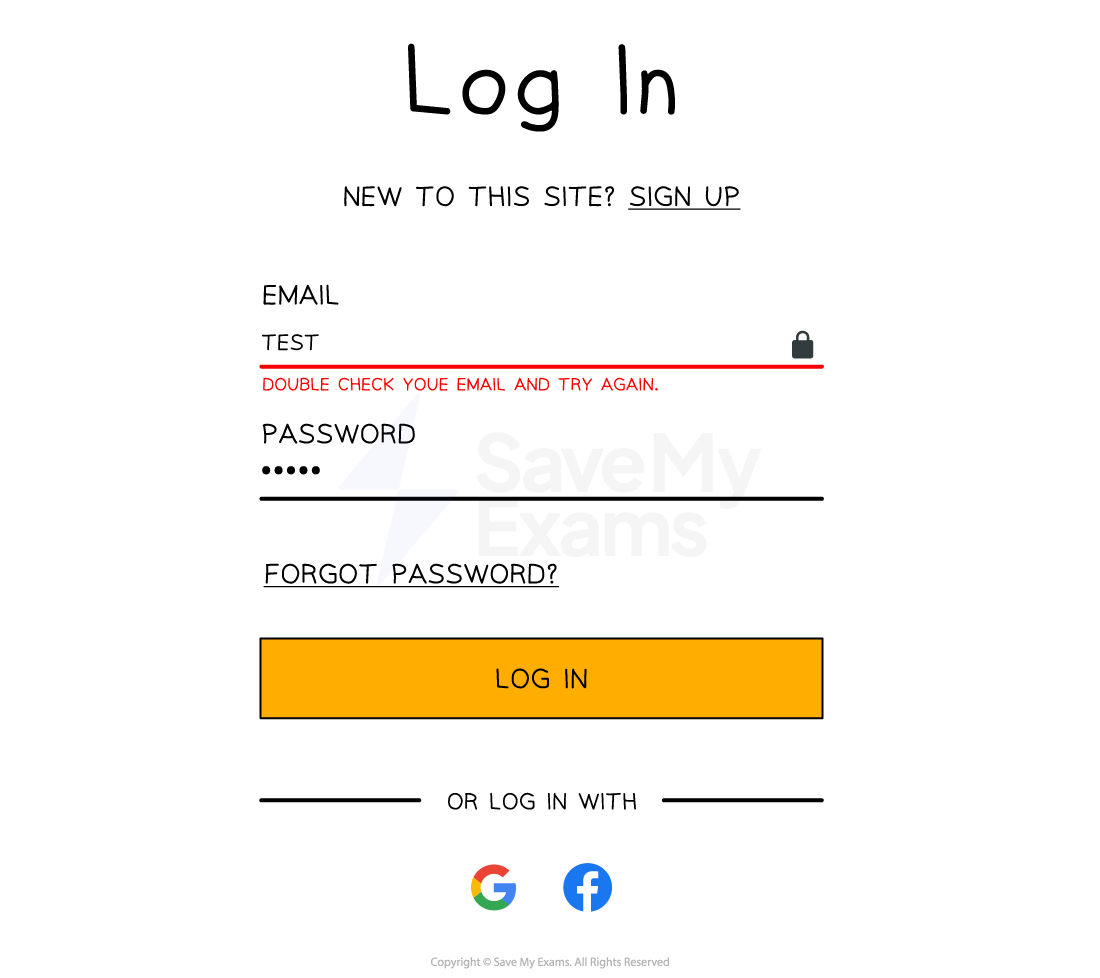
Length check
Checks the length of a string
An example is ensuring that a password is 8 or more characters in length
Code example
|
Range check
Ensures the data entered as a number falls within a particular range
An example is checking a user's age has been entered and falls between the digits of 0-100
Code example
|
Presence check
Looks to see if any data has been entered in a field
An example is checking that a user has entered a name when registering for a website
Code example
|
Pattern check
Ensures that the data has been entered in the correct pattern / format
An example would be ensuring that an email includes the @ symbol and a full stop (.)
Code example
|
This could be extended further and made a little more complex by ensuring that the data entered into a program follows the set format
In the example below, the user must enter a postcode in the format of LL00 0LL where L is a letter and 0 is a number
This example uses a length check and string slicing covered in the previous revision note
Code example
|
Worked Example
A car dealership uses a computer system to record details of the cars that it has for sale. Each car has a make, model, age and number of miles driven.
The car dealership only sells cars that have fewer than 15,000 miles and are 10 years old or less.
Write an algorithm that will:
Ask the user to enter the number of miles and the age of a car
Validate the input to check that only sensible values that are in the given range are entered
Output True if valid data has been entered or False if invalid data has been entered [4]
How to answer this question
When answering any algorithm question, ask yourself:
What inputs and outputs do I need?
Do I need to do any calculations or comparisons?
Do I need to use selection or iteration?
Do I need to use a function or procedure?
Re-read the algorithm question working through the criteria given
Programming Skill | Algorithm |
---|---|
Inputs |
|
Outputs |
|
Calculations / Comparisons |
|
Selection or Iteration |
|
Function or Procedure |
|
Answer: i)1 mark per bullet, max 4
Miles and age input separately
Checks for valid mileage
Checks for valid age
Checks both are greater than / greater than equal to zero
…correctly outputs both True and False
Example Answer:
miles = int(input("enter miles driven"))
age = int(input("enter age of car"))
valid = True
if miles > 15000 or miles < 0 then
valid = False
elif age > 10 or age < 0 then
valid = False
endif
print(valid)
You've read 0 of your 5 free revision notes this week
Sign up now. It’s free!
Did this page help you?