Stacks (OCR A Level Computer Science): Revision Note
Exam code: H446
Implementing a Stack
How Do You Program a Stack?
To recap the main operations of Stacks and how they work, follow this link
When programming a stack, the main operations listed below must be included in your program.
The main operations for Stacks are:
Main Operations
Operation | Python | Java |
---|---|---|
isEmpty() |
|
|
push(value) |
|
|
peek() |
|
|
pop() |
|
|
size() |
|
|
isFull() |
|
|
Programming a Stack in Python
Explanation of the Python Code
Class Stack: Defines a Python class, Stack, with a specified capacity and an empty list to store elements.
Push Method: Appends an item to the stack if the stack is not full; otherwise, it prints a message to say that it is not possible.
Pop Method: Removes and returns the top item from the stack if it's not empty; otherwise, it prints a message to say nothing can be popped as it is empty.
Peek Method: Returns the top item of the stack without removing it if the stack is not empty; otherwise, it will print a message to say the stack is empty.
Additional Operations: The code at the bottom includes methods to retrieve the stack size (size), check if the stack is empty (isEmpty), and check if the stack is full (isFull).
class Stack:
def __init__(self, capacity):
self.capacity = capacity
self.stack = []
def push(self, item):
if len(self.stack) < self.capacity:
self.stack.append(item)
print("Pushed item:", item)
else:
print("Stack is full. Cannot push item:", item)
def pop(self):
if not self.isEmpty():
return self.stack.pop()
else:
print("Stack is empty. Cannot pop item.")
def peek(self):
if not self.isEmpty():
return self.stack[-1]
else:
print("Stack is empty. Cannot peek.")
def size(self):
return len(self.stack)
def isEmpty(self):
return len(self.stack) == 0
def isFull(self):
return len(self.stack) == self.capacity
This code below is used to demonstrate the usage of the operations above with a stack of animals, pushing, popping, peeking, and checking the stack's status.
# Create a stack with a capacity of 5
stack = Stack(5)
# Push animals onto the stack
stack.push("Dog")
stack.push("Cat")
stack.push("Elephant")
stack.push("Lion")
stack.push("Tiger")
# Try pushing more animals than the capacity allows
stack.push("Giraffe") # Output: Stack is full. Cannot push item: Giraffe
# Pop an animal from the stack
popped_animal = stack.pop()
print("Popped animal:", popped_animal) # Output: Popped animal: Tiger
# Peek at the top animal of the stack
top_animal = stack.peek()
print("Top animal:", top_animal) # Output: Top animal: Lion
# Get the size of the stack
stack_size = stack.size()
print("Stack size:", stack_size) # Output: Stack size: 4
# Check if the stack is empty
is_empty = stack.isEmpty()
print("Is stack empty?", is_empty) # Output: Is stack empty? False
# Check if the stack is full
is_full = stack.isFull()
print("Is stack full?", is_full) # Output: Is stack full? False
Programming a Stack in Java
Explanation of the Java Code
AnimalStack Class: Defines a class named AnimalStack that uses the Stack class to implement a stack for storing animal names with a specified capacity.
Push Method: Adds an animal to the stack if it's not full; otherwise, it prints a message to say that it is not possible.
Pop Method: Removes and returns the top animal from the stack if it's not empty; otherwise, it prints a message to say that it is not possible and returns null.
Peek Method: Returns the top animal without removing it if the stack is not empty; otherwise, it prints a message to say that it is not possible and returns null.
Main Method Usage: Demonstrates the usage of the AnimalStack class with a stack of animals, showcasing pushing, popping, peeking, and checking stack size and status.
import java.util.Stack;
public class AnimalStack {
private int capacity;
private Stack<String> stack;
public AnimalStack(int capacity) {
this.capacity = capacity;
this.stack = new Stack<>();
}
public void push(String animal) {
if (stack.size() < capacity) {
stack.push(animal);
System.out.println("Pushed item: " + animal);
} else {
System.out.println("Stack is full. Cannot push item: " + animal);
}
}
public String pop() {
if (!isEmpty()) {
return stack.pop();
} else {
System.out.println("Stack is empty. Cannot pop item.");
return null;
}
}
public String peek() {
if (!isEmpty()) {
return stack.peek();
} else {
System.out.println("Stack is empty. Cannot peek.");
return null;
}
}
public int size() {
return stack.size();
}
public boolean isEmpty() {
return stack.isEmpty();
}
public boolean isFull() {
return stack.size() == capacity;
}
public static void main(String[] args) {
AnimalStack stack = new AnimalStack(5);
stack.push("Dog");
stack.push("Cat");
stack.push("Elephant");
stack.push("Lion");
stack.push("Tiger");
stack.push("Giraffe"); // Output: Stack is full. Cannot push item: Giraffe
String poppedAnimal = stack.pop();
System.out.println("Popped animal: " + poppedAnimal); // Output: Popped animal: Tiger
String topAnimal = stack.peek();
System.out.println("Top animal: " + topAnimal); // Output: Top animal: Lion
int stackSize = stack.size();
System.out.println("Stack size: " + stackSize); // Output: Stack size: 4
boolean isEmpty = stack.isEmpty();
System.out.println("Is stack empty? " + isEmpty); // Output: Is stack empty? false
boolean isFull = stack.isFull();
System.out.println("Is stack full? " + isFull); // Output: Is stack full? false
}
}
Ready to test your students on this topic?
- Create exam-aligned tests in minutes
- Differentiate easily with tiered difficulty
- Trusted for all assessment types
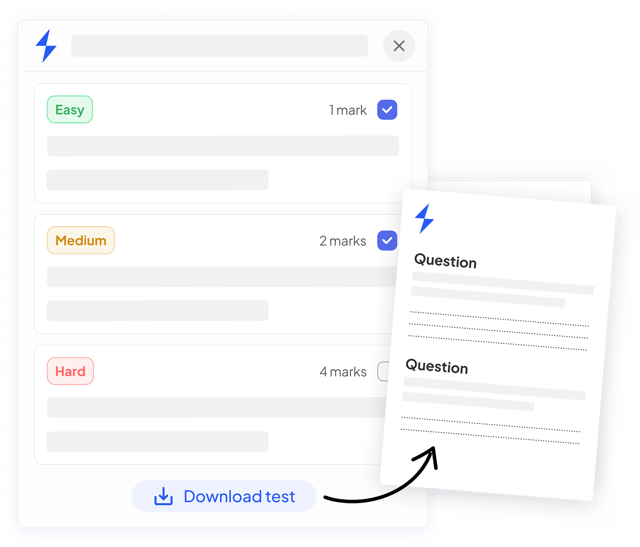
Did this page help you?