Queues (OCR A Level Computer Science): Revision Note
Exam code: H446
Implementing Linear Queues
How Do You Program a Queue?
To recap the main operations of Queues and how they work, follow this link
When programming a queue, the main operations listed below must be included in your program
Initialise the queue
Enqueue
Dequeue
Peek
Algorithm to Implement a Linear Queue
The code below initialises an array and index pointers to service a linear queue
The constant MAX_SIZE informs the size of the array
The front index pointer is initialised to 0 and the rear index pointer is initialised to -1
The rear index pointer will be incremented every time you add an item to the queue, so the initial value will ensure the first item is added into the first position of the array (index 0)
Once the first item has been added, both front and rear will correctly point to the item in the array
Pseudocode | Python | Java |
---|---|---|
|
|
|
EnQueue Data
Before adding an item to the queue you need to check that the queue is not full
When the rear index pointer is pointing to the final space in the array there is no room to add new items
A subroutine isFull() is used to carry out the check.
If the end of the array has not been reached, the rear index pointer is incremented and the new item is added to the queue
Pseudocode | Python | Java |
---|---|---|
|
|
|
DeQueue Data
Before taking an item from the queue you need to make sure that the queue is not empty
A subroutine isEmpty() can be defined for this purpose
The subroutine will check whether the front index pointer is ahead of the rear index pointer
If the queue is not empty the item at the front of the queue is returned and front is incremented by 1
Pseudocode | Python | Java |
---|---|---|
|
|
|
Implementing Circular Queues
Algorithm to Implement a Circular Queue
When you manipulate the items in a circular queue you must be able to advance the index pointers in such a way that they will reset to 0 once the end has been reached
(pointer + 1) MOD MAX_SIZE
This can be used to calculate the new position for the index pointer
Where the pointer is front or rear and max_size is the maximum number of items in the queue.
Let’s imagine there is an array with 7 items.
Initial Position | Pointer + 1 | (Pointer + 1) MOD 7 | New Position |
---|---|---|---|
0 | 1 | 1 | 1 |
1 | 2 | 2 | 2 |
2 | 3 | 3 | 3 |
3 | 4 | 4 | 4 |
4 | 5 | 5 | 5 |
5 | 6 | 6 | 6 |
6 | 7 | 0 | 0 |
When the index pointer references the last position in the array, position 6, the next position is calculated as (6+1) MOD 7 which gives the result 0
This allows the index pointer to move back to the reference at the start of the array
Initialise Circular Queue
The initialisation is the same as a linear queue apart from you initialise the front index to -1 as this will allow you to be certain that you have an empty queue
Pseudocode | Python | Java |
|
|
|
Enqueue Circular Queue
Before an item can be enqueued the array needs to be checked to see if it’s full
It will be full if the next position to be used is already occupied by the item at the front of the queue
If the queue is not full then the rear index pointer must be adjusted to reference the next free position so that the new item can be added
Pseudocode | Python | Java |
---|---|---|
|
|
|
DeQueue Circular Queue
Before dequeuing the array needs to be checked if it’s empty. If the queue is not empty then the item at the front is dequeued
If this is the only item that was in the queue, the rear and front pointers are reset
Otherwise the pointer moves to reference the next item in the queue
Pseudocode | Python | Java |
|
|
|
Implementing Priority Queues
Algorithm to Implement a Priority Queue
A priority queue is where each item in the queue has a priority
When new items are added, they are inserted ahead of those with a lower priority and behind items of equal priority
If you want to implement a priority queue it is best to use OOP
Encapsulating the data and methods within class definitions provides a neat solution and once written, the class can be reused in other applications that require a queue
Pseudocode |
ENDIF ENDFUNCTION |
Python |
|
Java |
|
Ready to test your students on this topic?
- Create exam-aligned tests in minutes
- Differentiate easily with tiered difficulty
- Trusted for all assessment types
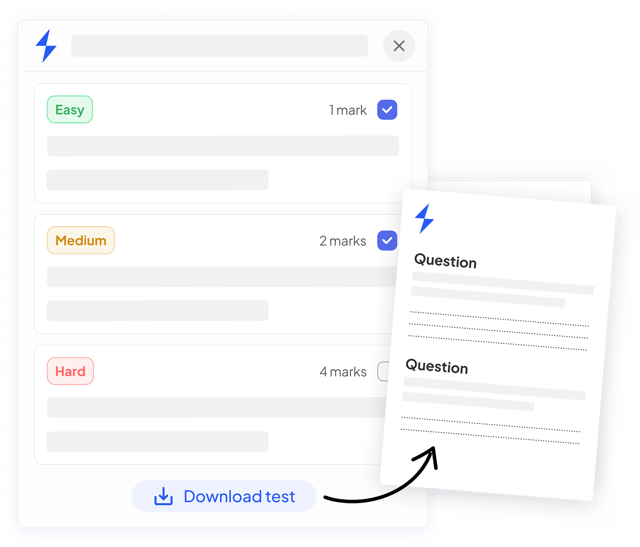
Did this page help you?