Selection (OCR A Level Computer Science)
Revision Note
Written by: Becci Peters
Reviewed by: James Woodhouse
Selection
What is Selection?
Selection is used within the branching (selection) programming construct
They are used to test conditions and the outcome of this condition will then determine which lines or block of code is run next
There are two ways to write selection statements:
if... elseif... else...
switch... case...
Writing a condition
Think of it like writing a yes/no question
For example
Is the number bigger than 10?
Is the number between 50 and 100?
Is the answer Paris?
If the question can’t be answered with yes/no then it needs to be rewritten in this way
Using more than one operator
Using one operator is most common but sometimes two are needed. More than two operations can be used but it gets more complicated as it involves using Boolean operators
Imagine a program where the user has to enter a number based on the role of a dice. The number needs to be between 1 and 6
The yes/no question could be is the number between 1 and 6 - but it would be structured slightly differently in the code
if number >=1 and number <=6 then
The first check is if the number is greater than or equal to 1
The second check is if the number is less than or equal to 6
The final check is if both sides are true
If Statements
An
if elseif else
statement will let you choose a line/lines of code to run if a condition is true or falseBelow are three ways to use
if
statements:
if...
if... else...
if... elseif... else...
Syntax of an if... statement
The syntax of an if
the statement consists of the if
keyword, followed by a condition, and a code block that is executed if the condition evaluates to true.
if condition then
// Code to be executed if the condition is true
endif
Pseudocode example
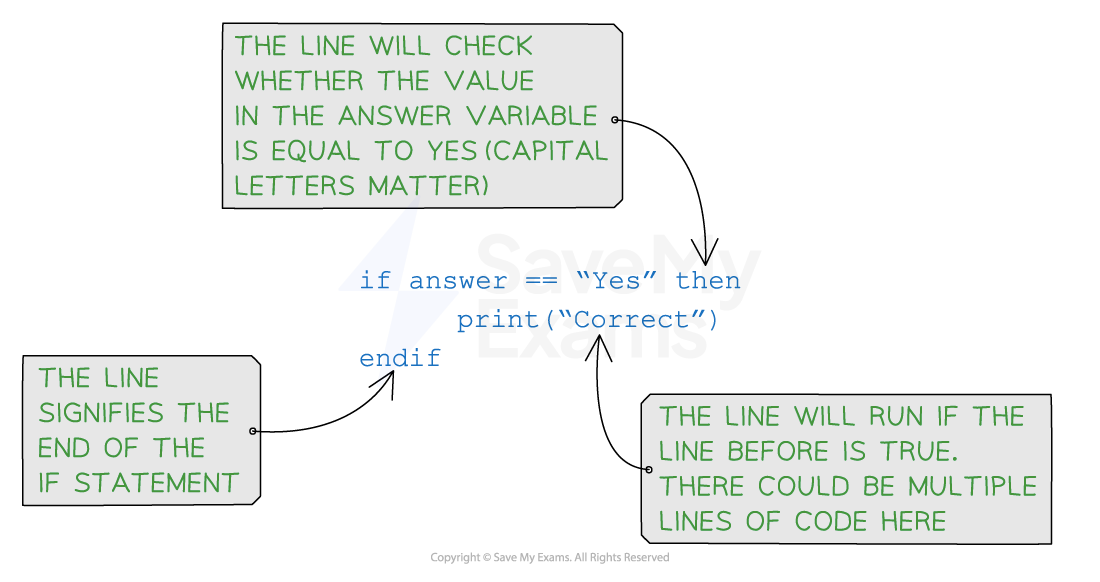
if
statement example pseudocode
Python example
number = 5
if number > 0:
print('The number is positive.')
In this example, the
if
statement checks if the value of the variablenumber
is greater than0
. If the condition is true, the message'The number is positive.'
is output
Java example
public class Main {
public static void main(String[] args) {
int number = 5;
if (number > 0) {
System.out.println("The number is positive.");
}
}
}
Syntax of an if... else... statement
The if
statement can be extended with an else
clause to specify an alternative block of code that is executed when the condition evaluates to false.
if condition then
// Code to be executed if the condition is true
else
// Code to be executed if the condition is false
endif
Pseudocode example
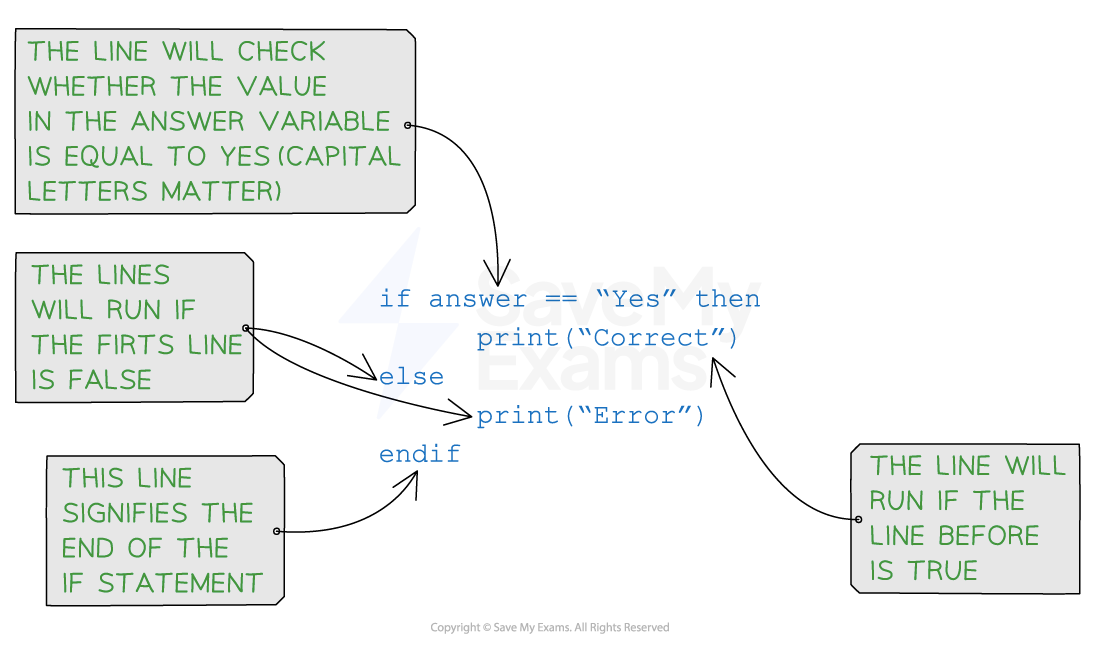
if else
statement example pseudocode
Python example
number = -3
if number > 0:
print('The number is positive.')
else:
print('The number is not positive.')
In this example, if the value of number
is greater than 0, the message 'The number is positive.'
is output. Otherwise, the message 'The number is not positive.'
is output.
Java example
public class Main {
public static void main(String[] args) {
int number = -3;
if (number > 0) {
System.out.println("The number is positive.");
} else {
System.out.println("The number is not positive.");
}
}
}
Syntax of an if... elseif... else... statement
The elseif
clause specifies additional conditions to check if the initial if
condition is false. This allows the handling of multiple scenarios in a more complex decision-making process:
if condition then
// Code to be executed if the condition is true
elseif condition then
// Code to be executed if the condition is true
else
// Code to be executed if all conditions are false
endif
Pseudocode example
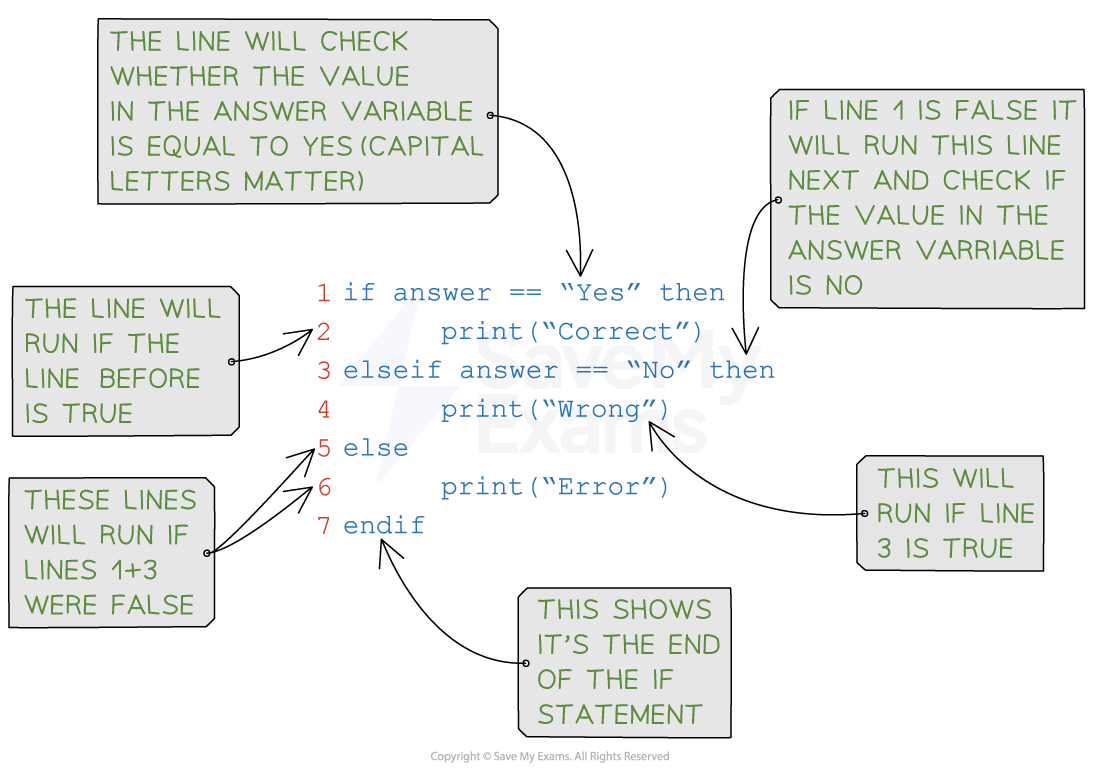
if elseif else
statement example pseudocode
Python example
score = 85
if score >= 90:
print('Excellent!')
elif score >= 80:
print('Good.')
elif score >= 70:
print('Fair.')
else:
print('Needs improvement.')
In this example, the if elseif else
statement evaluates the value of score
to determine the corresponding message based on the score range.
Java example
public class Main {
public static void main(String[] args) {
int score = 85;
if (score >= 90) {
System.out.println("Excellent!");
} else if (score >= 80) {
System.out.println("Good.");
} else if (score >= 70) {
System.out.println("Fair.");
} else {
System.out.println("Needs improvement.");
}
}
}
Examiner Tips and Tricks
You can use as many
elseif...
statements as you want to but it might be clearer to use aswitch case
statement.Check whether to use an
and
or anor
operator in the condition as it’s easy to get these mixed up. For example:if number <0 and number >100
will check if the number is both below 0 and greater than 100 (however a number cannot be both less than 0 and greater than 100)if number <0 or number >100
will check if the number is between 1 and 99 (this will check that the number is either less than 0 or greater than 100)
Worked Example
Two people play a counting game. The rules of the game are as follows:
• The first player starts at 1
• Each player may enter one, two or three numbers on their turn, and the numbers must be in ascending order
• Players take it in turns to choose
• The player who chooses “15” loses the game
For example, if the first player chooses three numbers (1, 2, 3) then the second player could choose one number (4), two numbers (4, 5) or three numbers (4, 5, 6). The first player then takes another go.
Write an algorithm using pseudocode that allows two players to play this game. The algorithm should:
• Alternate between player 1 and player 2
• Ask the player how many numbers they would like to choose, ensuring that this is between 1 and 3
• Display the numbers that the player has chosen
• Display a suitable message to say which player has won once the number 15 has been displayed
8 marks
How to answer this question:
We'll start by asking the player how many numbers they want to choose and check they've entered a number between 1 and 3. We'll do this as a loop which will keep asking them how many numbers until they've entered either 1, 2 or 3
choice=0
while choice < 1 or choice > 3:
choice = input("how many numbers?")
endwhile
Now we'll display the numbers the player has chosen. We'll need to set num=1 at the start of the code
for y = 1 to choice
print(num)
num = num + 1
next y
Now we'll make this code run until a player chooses 15
num = 1
while num <= 15
choice = 0
while choice < 1 or choice > 3
choice = input("how many numbers?")
endwhile
for y = 1 to choice
print(num)
num = num + 1
next y
endwhile
print(turn + " wins!")
Now we'll get the players to take turns by setting whose turn it is before the loop and then swapping whose go it is during the loop
turn = "player 1"
if turn == "player 1" then
turn = "player 2"
else
turn = "player 1"
endif
Answer:
num = 1
turn = "player 1"
while num <= 15
print(turn + "'s turn")
choice = 0
while choice < 1 or choice > 3
choice = input("how many numbers?")
endwhile
for y = 1 to choice
print(num)
num = num + 1
next y
//swap turn
if turn == "player 1" then
turn = "player 2"
else
turn = "player 1"
end if
endwhile
print(turn + " wins!")
Case Statements
switch/case
is a type of conditional statement that provides an alternative way to perform multiple comparisons based on the value of an expressionThese statements are particularly useful when you have a single expression that you want to compare against multiple possible values
Syntax of a switch case statement
The syntax of a
switch case
statement consists of theswitch
keyword followed by an expressionThis expression is evaluated, and its value is then compared against various
case
labels. If a match is found, the corresponding block of code is executedThe
default
keyword is optional and specifies a block of code to be executed if none of the case labels match the expression
switch expression
case value1:
// Code to be executed if the expression matches value1
case value2:
// Code to be executed if the expression matches value2
// more case statements if needed
default:
// Code to be executed if no case matches the expression
endswitch
Python example
grade='b'
match (grade):
case 'a':
print("Excellent")
case 'b':
print("Good")
case 'c':
print("Fair")
case_:
print("Needs improvement")
Java example
public class Main {
public static void main(String[] args) {
char grade = 'b';
switch (grade) {
case 'a':
System.out.println("excellent");
break;
case 'b':
System.out.println("good");
break;
case 'c':
System.out.println("fair");
break;
default:
System.out.println("needs improvement");
}
}
}
Last updated:
You've read 0 of your 5 free revision notes this week
Sign up now. It’s free!
Did this page help you?