Programming Inheritance (OCR A Level Computer Science)
Revision Note
Written by: Robert Hampton
Reviewed by: James Woodhouse
Programming Inheritance
To program inheritance, you should already have a solid understanding of what inheritance in Object Oriented Programming (OOP) is.
How do you Define Inheritance?
Pseudocode
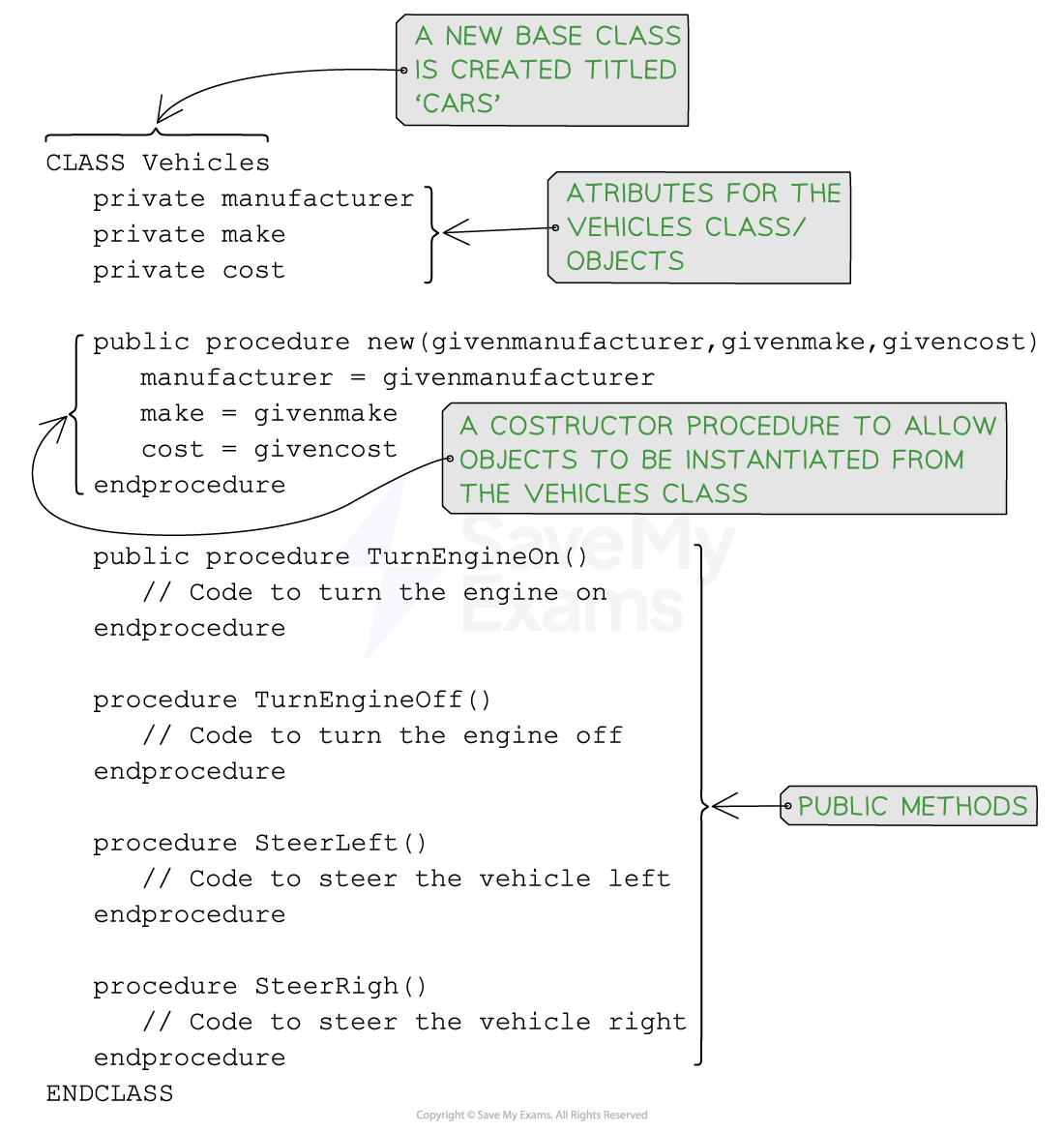
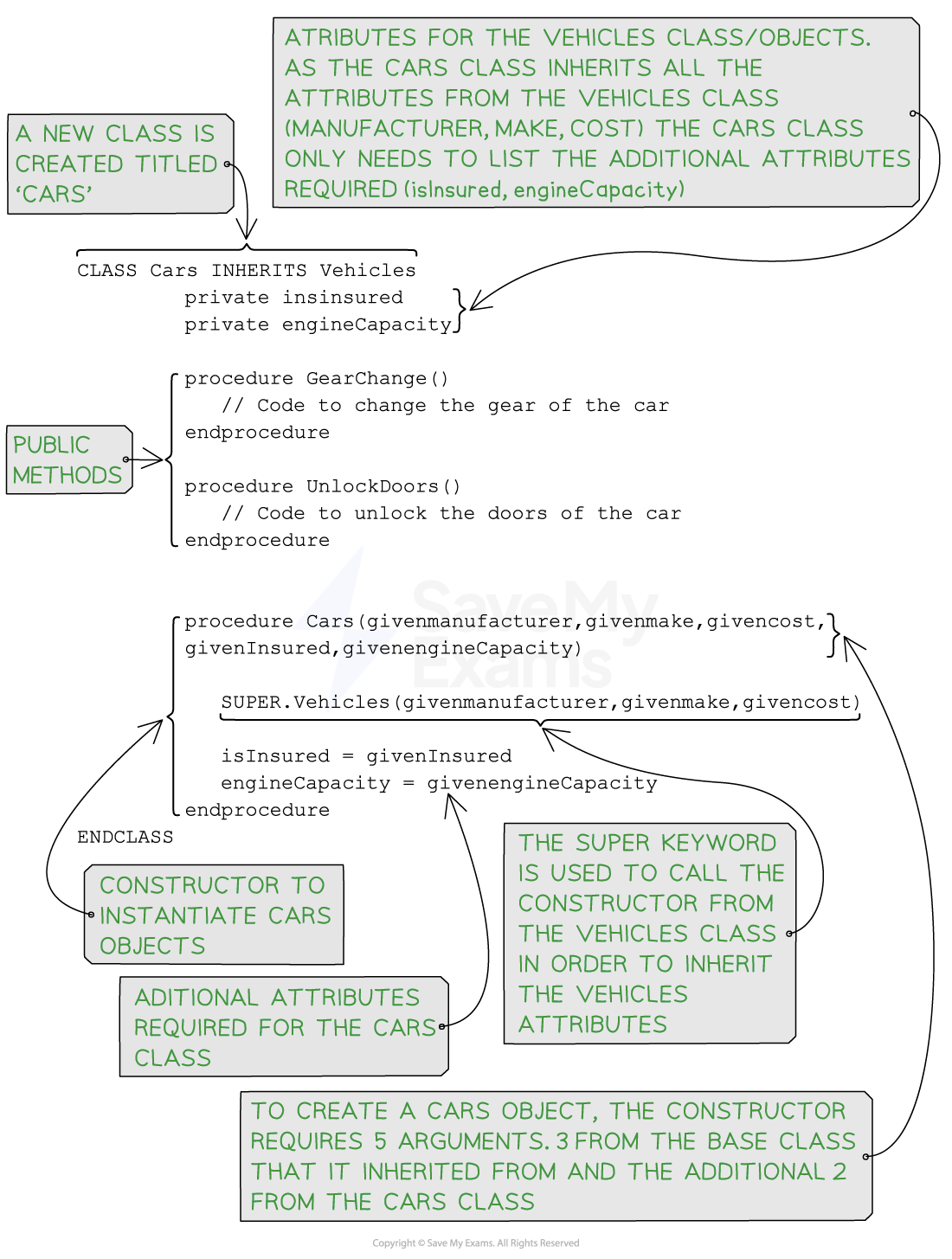
Pseudocode for a class created using inheritance
Java
//vehicle class created
public class Vehicle {
//attributes created for the vehicle class
private String manufacturer;
private String make;
private double cost;
//constructor to create objects of type vehicle
public Vehicle(String manufacturer, String make, double cost) {
this.manufacturer = manufacturer;
this.make = make;
this.cost = cost;
}
//method to start the engine of the car
public void turnEngineOn() {
//code to turn the engine on
}
//method to stop the engine of the car
public void turnEngineOff() {
//code to turn the engine off
}
//method to steer the car to the left
public void steerLeft() {
// code to steer the vehicle left
}
//method to steer the car to the right
public void steerRight() {
// code to steer the vehicle right
}
}
//Derived Class (Car)
//the Car class uses the keyword 'extends' to inherit from the Vehicle class
public class Car extends Vehicle {
private boolean isInsured;
private double engineCapacity;
// Constructor to create objects of type Car
public Car(String manufacturer, String make, double cost, boolean isInsured, double engineCapacity) {
//the super keyword is used to inherit the attributes from the base class
super(manufacturer, make, cost);
this.isInsured = isInsured;
this.engineCapacity = engineCapacity;
}
//method to change gear
public void gearChange() {
//code to change the gear of the car
}
//method to unlock the vehicle doors
public void unlockDoors() {
//code to unlock the doors of the car
}
}
Python
#Base class (Vehicle)
#Vehicle class created
class Vehicle:
#attributes created for the Vehicle class
def __init__(self, manufacturer, make, cost):
self.manufacturer = manufacturer
self.make = make
self.cost = cost
#method to start the engine of the car
def turn_engine_on(self):
# Code to turn the engine on
#method to stop the engine of the car
def turn_engine_off(self):
# Code to turn the engine off
#method to steer the car to the left
def steer_left(self):
# Code to steer the vehicle left
#method to steer the car to the right
def steer_right(self):
# Code to steer the vehicle right
#Derived Class (Car)
#the Car class uses the keyword parent class name in parenthesis to inherit from the Vehicle class
class Car(Vehicle):
def __init__(self, manufacturer, make, cost, is_insured, engine_capacity):
#the super keyword is used to inherit the attributes from the base class
super().__init__(manufacturer, make, cost)
self.is_insured = is_insured
self.engine_capacity = engine_capacity
#method to change gear
def gear_change(self):
# Code to change the gear of the car
#method to steer the car to the right
def unlock_doors(self):
# Code to unlock the doors of the car
Last updated:
You've read 0 of your 5 free revision notes this week
Sign up now. It’s free!
Did this page help you?