Modularity, Functions & Procedures (OCR A Level Computer Science) : Revision Note
Modularity
What is Modularity?
Modularity is a concept where problems are broken down into more manageable pieces
Each piece of the problem should be carried out by one single subroutine
Subroutines, also known as modules are standalone blocks of code and when called they will complete a task
They promote code reusability, modularity, and organisation, enabling a programmer to write efficient and maintainable programs
Functions and procedures are examples of subroutines and they are both very similar in nature
Difference between functions and procedures
Functions will return a value. For example if a function completes a calculation, then the result of the calculation will be returned to the part of the programming code that called the function
Procedures can also be called to complete a task, however, they do not return a value back to the part of the programming code that called the procedure
Both functions and procedures may need a parameter. These are pieces of data which are passed into the function or procedure to allow it to complete its task
Considerations and best practices
Naming: Choose descriptive and meaningful names for your functions and procedures that indicate their purpose
Parameter names: Use clear and meaningful parameter names to improve code readability
Focus: Aim for functions and procedures that are short and focused so they they complete a specific task
Return values: Functions should have explicit return statements with meaningful return values, while procedures should not have return statements
Functions
The syntax for defining a function is as follows:
function functionName(parameter1, parameter2)
// Code block to perform the task
// Return value;
endfunction
Pseudocode example
In the example below two numbers are passed as parameters (a and b) into the function which adds them together and returns the result.
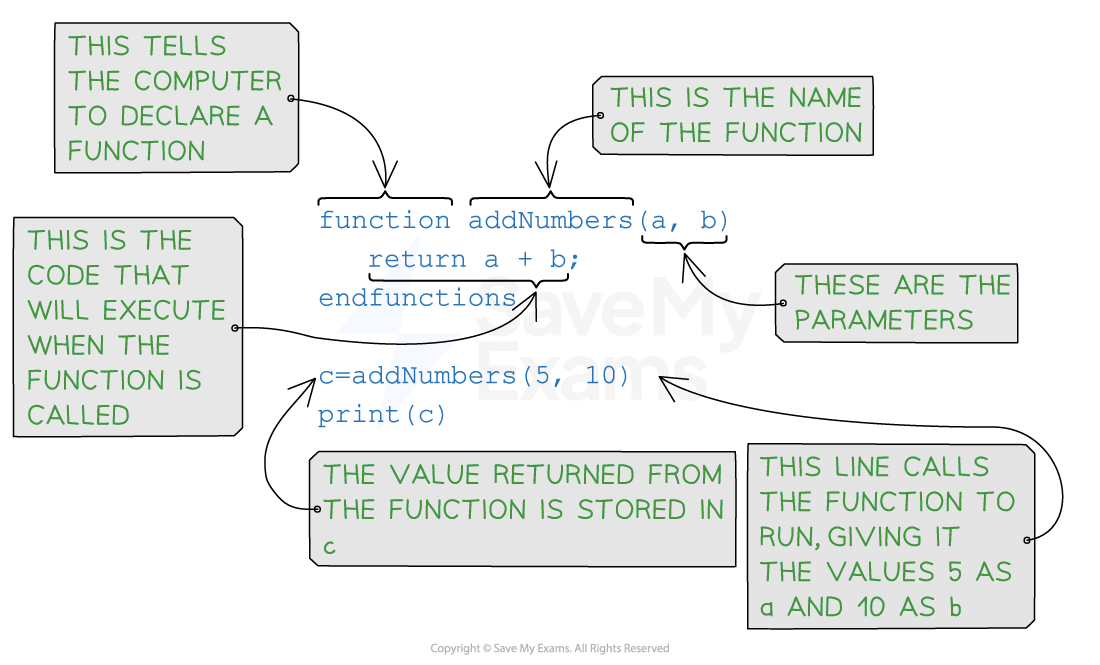
Function example in pseudocode
Python example
def add_numbers(a, b):
return a + b
c = add_numbers(5, 10)
print(c)
Java example
public class Main {
public static void main(String[] args) {
int c = addNumbers(5, 10);
System.out.println(c);
}
public static int addNumbers(int a, int b) {
return a + b;
}
}
Procedures
A procedure is similar to a function but does not return a value. Instead, it performs a series of actions or operations which could be anything the programmer wants the procedure to execute
A procedure is essentially a function without a
return
statement or with areturn
statement that has no value to return
The syntax is the same as for functions:
procedure procedureName(parameter1, parameter2)
// Code block to perform actions
// No return statement or return with no value;
endprocedure
Pseudocode example
In the example below two numbers are passed as parameters (a and b) into the procedure which adds them together and prints the result. As this is a procedure, the result cannot be returned.
procedure addNumbers (a,b)
total = a + b
print (total) // The total is printed rather than returned
endprocedure
c = addNumbers(5,10)
print (c)
You've read 0 of your 5 free revision notes this week
Sign up now. It’s free!
Did this page help you?