Translation Skills (Cambridge (CIE) A Level Computer Science) : Revision Note
Structured English/Flowchart to Pseudocode
Example - Structured English
A customer is buying an item. If the customer has a discount code, they receive 10% off the price. Otherwise, they pay the full amount. The program should ask for the item price and whether they have a discount code, then calculate and display the final price.
Step 1 - Structured English
Ask the user to enter the price of the item
Ask the user if they have a discount code
If they do have a discount code
→ Calculate 10% off the price
→ Subtract the discount from the original priceOtherwise
→ The price stays the sameOutput the final price
Step 2 – Identifier table
Identifier | Description |
---|---|
| Original price of the item |
| TRUE if user has a discount code |
| Amount taken off the original price |
| Price after discount is applied |
Step 3 – Pseudocode
// Ask for item price
INPUT ItemPrice
// Ask if user has a discount code
INPUT HasDiscount
IF HasDiscount = TRUE THEN
DiscountAmount ← ItemPrice * 0.10
FinalPrice ← ItemPrice - DiscountAmount
ELSE
FinalPrice ← ItemPrice
ENDIF
// Output the final price
OUTPUT "The final price is ", FinalPrice
Structured English/Pseudocode to Flowchart
Example - Pseudocode
INPUT Number
IF Number MOD 2 = 0 THEN
OUTPUT "Even"
ELSE
OUTPUT "Odd"
ENDIF
Step 1 – Identifier table
Identifier | Description |
---|---|
| The number entered by the user |
Step 2 – Flowchart structure
Pseudocode statement | Flowchart symbol | Purpose |
---|---|---|
| Parallelogram (Input/Output) | User enters a number |
| Diamond (Decision) | Checks if the number is divisible by 2 |
| Parallelogram (Input/Output) | Displays message if the condition is true |
| Parallelogram (Input/Output) | Displays message if the condition is false |
Start/End | Oval | Denotes beginning and end of the process |
Arrows | Lines with arrows | Indicate flow of control |
Step 4 - Flowchart
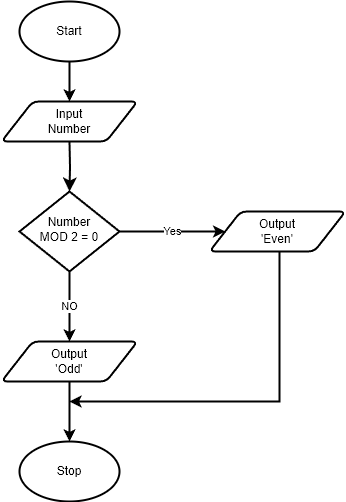
You've read 0 of your 5 free revision notes this week
Unlock more, it's free!
Did this page help you?